Introduction to Programming
Lecture No. 16
Reading Material
Deitel & Deitel - C++ How to Program Chapter 5, 18
5.9, 5.10, 18.4
Summary
· Pointers (continued)
· Multi-dimensional Arrays
· Pointers to Pointers
· Command-line Arguments
· Exercises
· Tips
Pointers (continued)
We will continue with the elaboration of the concept of pointers in this lecture. To further understand pointers, let's consider the following statement.
char myName[] = "Full Name";
This statement creates a 'char' type array and populates it with a string. Remember the character strings are null ( '\0' ) terminated. We can achieve the same thing with the use of pointer as under:
char * myNamePtr = "Full Name";
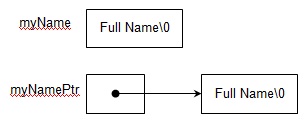
Let's see what's the difference between these two approaches?
When we create an array, the array name, 'myName' in this case, is a constant pointer. The starting address of the memory allocated to string "FullName" becomes the contents of the array name 'myName' and the array name 'myName' can not be assigned any other value. In other words, the location to which array names points to can not be changed. In the second statement, the 'myNamePtr' is a pointer to a string "FullName", which can always be changed to point to some other string.
Hence, the array names can be used as pointers but only as constant ones.
Multi-dimensional Arrays
Now we will see what is the relationship between the name of the array and the pointer. Suppose we have a two-dimensional array:
char multi[5][10];
In the above statement, we have declared a 'char' type array of 5 rows and 10 columns.
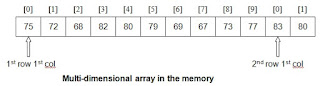
As discussed above, the array name points to the starting memory location of the memory allocated for the array elements. Here the question arises where the 'multi' will be pointing if we add 1 to ‘multi’.
We know that a pointer is incremented by its type number of bytes. In this case, 'multi' is an array of 'char' type that takes 1 byte. Therefore, ‘muti+1’ should take us to the second element of the first row (row 0). But this time, it is behaving differently. It is pointing to the first element (col 0) of the second row (row 1). So by adding '1' in the array name, it has jumped the whole row or jumped over as many memory locations as number of columns in the array. The width of the columns depends upon the type of the data inside columns. Here, the data type is 'char', which is of 1 byte. As the number of columns for this array 'multi' is 10, it has jumped 10 bytes.
Remember, whenever some number is added in an array name, it will jump as many rows as the added number. If we want to go to the second row (row 1) and third column (col 2) using the same technique, it is given ahead but it is not as that straight forward. Remember, if the array is to be accessed in random order, then the pointer approach may not be better than array indexing.
We already know how to dereference array elements using indexing. So the element at second row and third column can be accessed as 'multi[1][2]'.
To do dereferencing using pointers we use '*' operator. In case of one-dimensional array, '*multi' means 'the value at the address, pointed to by the name of the array'. But for two-dimensional array '*multi' still contains an address of the first element of the first row of the array or starting address of the array 'multi'. See the code snippet to prove it.
/* This program uses the multi-dimensional array name as
pointer */
#include <iostream.h>
void main(void)
{
//To
avoid any confusion, we have used ‘int’ type below
int multi[5][10];
cout
<< "\n The value of multi is: " << multi;
cout
<< "\n The value of *multi is: " << *multi;
}
|
Now, look at the output below:
The value of multi is: 0x22feb0
The value of *multi is: 0x22feb0
|
It is pertinent to note that in
the above code, the array ‘multi’ has been changed to ‘int’ from ‘char’ type to
avoid any confusion.
To access the elements of the
two-dimensional array, we do double dereferencing like '**multi'. If we want to
go to, say, 4th row (row 3), it is achieved as 'multi + 3' . Once reached in the
desired row, we can dereference to go to the desired column. Let's say we want
to go to the 4th column (col 3). It can be done in the following manner.
*(*(multi+3)+3)
This is an alternative way of
manipulating arrays. So 'multi[3][3]' element can also be accessed by
'*(*(multi+3)+3)'.
There is another alternative of
doing this by using the normal pointer. Following code reflects it.
/* This program uses array manipulation using indexing */
#include <iostream.h>
void main(void)
{
int multi [5][10];
int *ptr; // A normal ‘int’ pointer
ptr = *multi; // ‘ptr’ is assigned the starting
address of the first row
/* Initialize
the array elements */
for(int i=0; i
< 5; i++)
{
for (int
j=0; j < 10; j++)
{
multi[i][j] = i * j;
}
}
/* Array
manipulation using indexing */
cout <<
"\n Array manipulated using indexing is: \n";
for(int i=0; i
< 5; i++)
{
for (int
j=0; j < 10; j++)
{
cout
<< multi[i][j] << '\t';
}
cout
<< '\n';
}
/* Array
manipulation using pointer */
cout <<
"\n Array manipulated using pointer is: \n";
for(int k=0; k
< 50; k++, ptr ++) //
5 * 10 = 50
{
cout
<< *ptr << '\t';
}
}
|
The
output of this program is:
Array manipulated using indexing is:
0 0 0
0 0 0
0 0 0
0
0 1 2
3 4 5
6 7 8
9
0 2 4
6 8 10
12 14 16
18
0 3 6
9 12 15
18 21 24
27
0 4 8
12 16 20
24 28 32
36
Array manipulated
using pointer is:
0 0 0
0 0 0
0 0 0
0 0 1
2 3 4
5 6 7
8 9 0
2 4 6
8 10 12
14 16 18
0 3 6
9 12 15
18 21 24
27 0 4
8 12 16
20 24 28
32 36
|
The above line of output of array
manipulation is wrapped because of the fixed width of the table. Actually, it
is a single line.
Why it is a single line? As
discussed in the previous lectures, computer stores array in straight line
(contiguous memory locations). This straight line is just due to the fact that
a function accepting a multi-dimensional array as an argument, needs to know
all the dimensions of the array except the leftmost one. In case of
two-dimensional array, the function needs to know the number of columns so that
it has much information about the end and start of rows within an array.
It is recommended to write
programs to understand and practice the concepts of double dereferencing,
single dereferencing, incrementing the name of the array to access different
rows and columns etc. Only hands on practice will help understand the concept
thoroughly.
Pointers to Pointers
What we have been talking about,
now we will introduce a new terminology, is actually a case of ‘Pointer to
Pointer’. We were doing double dereferencing to access the elements of a
two-dimensional array by using array name (a pointer) to access a row (another
pointer) and further to access a column element (of ‘int’ data type).
In case of single dereference,
the value of the pointer is the address of the variable that contains the value
desired as shown in the following figure. In the case of pointer to pointer or
double dereference, the first pointer contains the address of the second
pointer, which contains the address of the variable, which contains the desired
value.
0 comments:
Post a Comment